- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.

Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Similar Reads
- Different Forms of Assignment Statements in Python We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object. There are some important properties of assignment in Python :- 3 min read
- Python | Priority key assignment in dictionary Sometimes, while working with dictionaries, we have an application in which we need to assign a variable with a single value that would be from any of given keys, whichever occurs first in priority. Let's discuss certain ways in which this task can be performed. Method #1 : Using loop This task can 4 min read
- Provide Multiple Statements on a Single Line in Python Python is known for its readability and simplicity, allowing developers to express concepts concisely. While it generally encourages clear and straightforward code, there are scenarios where you might want to execute multiple statements on a single line. In this article, we'll explore the logic, and 3 min read
- Difference between List VS Set VS Tuple in Python dynamic-sizedList: Lists are just like dynamic-sized arrays, declared in other languages (vector in C++ and ArrayList in Java). Lists need not be homogeneous always which makes it the most powerful tool in Python. The main characteristics of lists are - The list is a datatype available in Python whi 3 min read
- Difference Between Integer and Float in Python Integers are used to represent whole numbers without any decimal points, floats, or floating-point numbers, accommodate values with decimal places. Understanding the differences between these data types is important for effective programming and data manipulation in Python. In this article, we will 3 min read
- Add Same Key in Python Dictionary In Python, dictionaries are a versatile data structure that allows you to store key-value pairs. If we want to add the same key with a new value to a dictionary, there are multiple methods to achieve this. In this discussion, we will explore different methods for adding the same key with a new value 3 min read
- Python | Min and Max value in list of tuples The computation of min and max values is a quite common utility in any programming domain be it development or any other field that includes any programming constructs. Sometimes, data can come in the format of tuples and min and max operations have to be performed in them. Let's discuss certain way 7 min read
- Append Elements to Empty List in Python In Python, lists are used to store multiple items in one variable. If we have an empty list and we want to add elements to it, we can do that in a few simple ways. The simplest way to add an item in empty list is by using the append() method. This method adds a single element to the end of the list. 2 min read
- Python | Subtraction of dictionaries Sometimes, while working with dictionaries, we might have a utility problem in which we need to perform elementary operations among the common keys of dictionaries in Python. This can be extended to any operation to be performed. Let's discuss the subtraction of like key values and ways to solve it 6 min read
- Add Values into Empty List Using For Loop - Python Lists are versatile data structures that allow you to store and manipulate collections of items. The simplest way to add values to an empty list is by using append() method. This method adds a single item to the end of the list. [GFGTABS] Python a = [] # Loop through a range of numbers and add them 3 min read
- How to Update a Dictionary in Python This article explores updating dictionaries in Python, where keys of any type map to values, focusing on various methods to modify key-value pairs in this versatile data structure. Update a Dictionary in PythonBelow, are the approaches to Update a Dictionary in Python: Using with Direct assignmentUs 3 min read
- Iterate over characters of a string in Python In this article, we will learn how to iterate over the characters of a string in Python. There are several methods to do this, but we will focus on the most efficient one. The simplest way is to use a loop. Let’s explore this approach. Using for loopThe simplest way to iterate over the characters in 2 min read
- Python Program to Re-assign a dictionary based on path relation Given a dictionary, the task is to formulate a python program to re-assign it using a path relation among its keys and values, i.e value of one key is key to another. Example: Input : test_dict = {3 : 4, 5 : 6, 4 : 8, 6 : 9, 8 : 10} Output : {3: 10, 5: 9, 4: 10, 6: 9, 8: 10} Explanation : key 3 has 4 min read
- Accessing Python Function Variable Outside the Function In Python, variables defined within a function have local scope by default. But to Access function variables outside the function usually requires the use of the global keyword, but can we do it without using Global. In this article, we will see how to access a function variable outside the function 4 min read
- Python | Accessing variable value from code scope Sometimes, we just need to access a variable other than the usual way of accessing by it's name. There are many method by which a variable can be accessed from the code scope. These are by default dictionaries that are created and which keep the variable values as dictionary key-value pair. Let's ta 3 min read
- Starred Expression in Python In Python, the starred expression is a feature that allows for the unpacking of elements from iterable like lists. This feature is particularly useful when the number of elements is variable or when working with function arguments. One common use case of the starred expression is to unpack elements 3 min read
- Python | Assign multiple variables with list values We generally come through the task of getting certain index values and assigning variables out of them. The general approach we follow is to extract each list element by its index and then assign it to variables. This approach requires more line of code. Let's discuss certain ways to do this task in 4 min read
- How to add values to dictionary in Python In this article, we will learn what are the different ways to add values in a dictionary in Python. Assign values using unique keysAfter defining a dictionary we can index through it using a key and assign a value to it to make a key-value pair. C/C++ Code veg_dict = {} veg_dict[0] = 'Carrot' veg_di 3 min read
- How Can Python Lists Transformed into other Data Structures Python, a versatile and widely used programming language, provides a rich set of data structures to accommodate various programming needs. One of the fundamental data structures in Python is the list, a dynamic array that allows the storage of heterogeneous elements. While lists are powerful, there 4 min read
- Python Programs
- python-basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
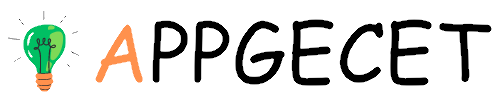
What is an Assignment Statement: Explained with Examples
- by Jackie Hobbs
- October 23, 2023
Welcome to our blog post on assignment statements ! If you’re new to programming or just dipping your toes into the world of coding, you may be wondering what exactly an assignment statement is. Simply put, an assignment statement is a fundamental concept in programming that allows us to assign values to variables.
In this blog post, we will explore what assignment statements are, how they work, and why they are essential in programming. Whether you’re a beginner or an experienced programmer, this post will provide you with a solid understanding of assignment statements using examples and real-world applications .
So, if you’re ready to dive into the world of assignment statements and discover the power they hold, let’s get started!
What is an Assignment Statement? Explain with an Example
An assignment statement is a fundamental concept in programming that involves giving a value to a variable. In simpler terms, it’s like giving a name to a particular storage space in the computer’s memory and storing something in it. Let’s dive deeper and explore this concept with an example!
Declaring Variables
Before we jump into assignment statements, let’s talk about variable declaration. In programming, variables are like containers used to store data. Think of them as labeled boxes where you can keep different things.
To declare a variable, you typically use syntax like this:
python num_apples = 5
Here, we declared a variable called num_apples and assigned it the value of 5 . Now, every time we refer to num_apples in our code, it will represent the value 5 .
The Assignment Statement
Now that we have our variable, let’s explore assignment statements. An assignment statement is used to update or change the value of a variable.
Imagine we’re in a magical universe where apples multiply themselves overnight. We can update the value of num_apples using an assignment statement like this:
python num_apples = num_apples * 2
This statement takes the current value of num_apples , which is 5 , multiplies it by 2 , and reassigns the result back to num_apples . So now, num_apples becomes 10 .
Using Assignment Statements in Real-Life Scenarios
Assignment statements are incredibly versatile and are used in numerous programming scenarios. Let’s explore a couple of examples to understand their practical applications better.
Example 1: Calculating the Area of a Rectangle
Imagine you’re writing a program to calculate the area of a rectangle. You can start by assigning values to the length and width variables:
python length = 10 width = 5
Next, you can use an assignment statement to calculate the area by multiplying the length and width:
python area = length * width
And there you have it! The variable area now holds the value 50 , which represents the calculated area of the rectangle.
Example 2: Simple String Manipulation
Assignment statements are not limited to numbers; they work with other data types too. Let’s say you want to create a greeting message by combining two strings:
python first_name = “John” last_name = “Doe”
By using the concatenation operator ( + ), you can combine the two strings and assign the result to a new variable called greeting :
python greeting = “Hello, ” + first_name + ” ” + last_name + “!”
Now, greeting holds the value "Hello, John Doe!" . Isn’t that neat?
Assignment statements are essential in programming as they allow us to store and update data in variables. By using them, we can manipulate values, perform calculations, and create dynamic programs. So embrace the magic of assignment statements and let your code come to life!
Happy coding in 2023 and beyond! 🚀
Frequently Asked Questions (FAQ) about Assignment Statements
What is an assignment.
An assignment in programming refers to the process of giving a value to a variable. It allows us to store and manipulate data in computer memory. In simpler terms, it’s like giving a name to a storage location in our code and putting something inside it.
How many types of assignment operators are there
In programming, we have several assignment operators. The most commonly used one is the equal sign (=), which assigns a value to a variable. However, there are also compound assignment operators, such as +=, -=, *=, and /=, which combine an operation and assignment in a single step.
What is the assignment operator
The assignment operator (=) is used to assign a value to a variable. It takes the value on the right side and stores it in the variable on the left side. It’s like playing the role of Santa Claus, delivering a gift to a variable and making it jolly.

What are assignment statements in Python
In Python, assignment statements are used to assign values to variables. They consist of the variable name, the assignment operator, and the value that we want to assign. For example, x = 5 assigns the value 5 to the variable named ‘x’.
What are the characteristics of a good assignment
A good assignment statement should be clear, meaningful, and accurate. It should use variable names that are descriptive and convey the purpose of the stored value. It’s like giving a name to a puppy – you want it to be cute and reflect its personality!
What is the relationship between an assignment statement and an expression statement
An assignment statement is a type of statement that includes an expression. The expression on the right side of the assignment operator is evaluated, and its resulting value is assigned to the variable on the left side. So, while an assignment statement includes an expression, not all expression statements are assignment statements.
What is an assignment statement, explain with an example
An assignment statement is a line of code that assigns a value to a variable. For example, let’s say we have a variable called name , and we want to assign the value “John” to it. We would write: name = "John" . This assigns the value “John” to the variable name .
How do you structure an assignment
To structure an assignment, you need a variable name on the left side of the assignment operator, followed by the assignment operator (=), and then the value or expression you want to assign on the right side. For example, x = 10 assigns the value 10 to the variable x .
Can homework kill you
Well, homework might not be deadly, but it can certainly feel like it! Don’t worry, though – taking breaks, managing your time wisely, and seeking help when needed can make homework much more manageable. And remember, your mental well-being is important too!
What is an assignment operator? Give an example.
An assignment operator is used to assign a value to a variable. The most commonly used assignment operator is the equal sign (=). For example, x = 5 assigns the value 5 to the variable x . It’s like a diligent worker bee buzzing around, making sure the values are assigned correctly.
What is called i 5 assignment
Apologies, but I’m not familiar with the concept of an “i 5 assignment.” However, if you’re referring to assigning the value 5 to a variable named ‘i’, you would write i = 5 . That’s a standard assignment statement. If there’s a particular concept or context you’re referring to, please provide more details!
What makes an assignment valid
An assignment is considered valid when it follows the syntax rules of the programming language and assigns a value to a variable in a correct and meaningful way. It should use valid variable names, proper assignment operators, and have a well-formed expression or value on the right side of the operator.
What are the 5 types of writing
The five main types of writing are:
- Narrative: Tells a story or recounts events.
- Descriptive: Paints a vivid picture using sensory details.
- Expository: Explains or informs about a specific topic.
- Persuasive: Convinces or argues for a particular position.
- Creative: Expresses original ideas and explores imagination.
Each type of writing serves different purposes and requires its own unique approach and style.
How do you start an assignment
To start an assignment, begin by understanding the task at hand. Read the instructions carefully and make sure you grasp the requirements. Next, brainstorm ideas, create an outline, and gather relevant information. Once you have a clear plan, you can start writing! Remember to break the task down into manageable chunks and set realistic deadlines to stay organized.
What does “!=” mean in Python
In Python, “!=” is the inequality operator. It checks if two values are not equal. For example, 5 != 10 evaluates to True because 5 is not equal to 10. Think of “!=” as a little detective that compares values and tells you if they’re different.
Stay tuned for more FAQ-style content and keep exploring the fascinating world of programming! Happy coding!
- assignment statements
- concatenation operator
- expression statement
- fundamental concept
- greeting message
Jackie Hobbs
The chemical composition of cng and its role in the automotive industry, battered vs. breaded: decoding the crispy coating conundrum, you may also like, is lead a reactive metal.
- October 10, 2023
Does Water or Alcohol Have a Higher Surface Tension?
- by PatrickTurner
- October 29, 2023
How Many WW Points is Half and Half?
- by Lindsey Smith
- October 22, 2023
Short Technology Cycles: Unraveling the Factors Behind the Rapid Evolution
- by Adam Davis
What is Land Use Pattern and Its Examples?
- by Erin Fuentes
How to Master Chapter 4 in Quantitative Research
- October 30, 2023
- Intro to Programming Logic >
Assignment Statements
Assignment statements in a program come in two forms – with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. Assignments with mutation are variable assignments that use the old value of a variable to calculate a value for the variable.
For example, an increment statement like x = x + 1 MUTATES the value of x by updating its value to be one bigger than it was before. In order to make sense of such a statement, we need to know the previous value of x .
In contrast, a statement like y = x + 1 assigns to y one more than the value in x . We do not need to know the previous value of y , as we are not using it in the assignment statement. (We do need to know the value of x ).
Assignments without mutation
We have already seen the steps necessary to process assignment statements that do not involve variable mutation. Recall that we can declare as a Premise any assignment statement or claim from a previous proof block involving variables that have not since changed.
For example, suppose we want to verify the following program so the assert statement at the end will hold (this example again eliminates the Logika mode notation and the necessary import statements, which we will continue to do in subsequent examples):
Since none of the statements involve variable mutation, we can do the verification in a single proof block:
Note that we did need to do AndI so that the last claim was y == z ∧ y == 6 , even though we had previously established the claims y == z and y == 6 . In order for an assert to hold (at least until we switch Logika modes in chapter 10), we need to have established EXACTLY the claim in the assert in a previous proof block.
Assignments with mutation
Assignments with mutation are trickier – we need to know the old value of a variable in order to reason about its new value. For example, if we have the following program:
Then we might try to add the following proof blocks:
…but then we get stuck in the second proof block. There, x is supposed to refer to the CURRENT value of x (after being incremented), but both our attempted claims are untrue. The current value of x is not one more than itself (this makes no sense!), and we can tell from reading the code that x is now 5, not 4.
To help reason about changing variables, Logika has a special Old(varName) function that refers to the OLD value of a variable called varName , just before the latest update. In the example above, we can use Old(x) in the second proof block to refer to x ’s value just before it was incremented. We can now change our premises and finish the verification as follows:
By the end of the proof block following a variable mutation, we need to express everything we know about the variable’s current value WITHOUT using the Old terminology, as its scope will end when the proof block ends. Moreover, we only ever have one Old value available in a proof block – the variable that was most recently changed. This means we will need proof blocks after each variable mutation to process the changes to any related facts.
Variable swap example
Suppose we have the following program:
We can see that this program gets two user input values, x and y , and then swaps their values. So if x was originally 4 and y was originally 6, then at the end of the program x would be 6 and y would be 4.
We would like to be able to assert what we did – that x now has the original value from y , and that y now has the original value from x . To do this, we might invent dummy constants called xOrig and yOrig that represent the original values of those variables. Then we can add our assert:
We can complete the verification by adding proof blocks after assignment statements, being careful to update all we know (without using the Old value) by the end of each block:
Notice that in each proof block, we express as much as we can about all variables/values in the program. In the first proof block, even though xOrig and yOrig were not used in the previous assignment statement, we still expressed how the current values our other variables compared to xOrig and yOrig . It helps to think about what you are trying to claim in the final assert – since our assert involved xOrig and yOrig , we needed to relate the current values of our variables to those values as we progressed through the program.
Last modified by: Julie Thornton Apr 25, 2024
Variable Assignment
To "assign" a variable means to symbolically associate a specific piece of information with a name. Any operations that are applied to this "name" (or variable) must hold true for any possible values. The assignment operator is the equals sign which SHOULD NEVER be used for equality, which is the double equals sign.
The '=' symbol is the assignment operator. Warning, while the assignment operator looks like the traditional mathematical equals sign, this is NOT the case. The equals operator is '=='
Design Pattern
To evaluate an assignment statement:
- Evaluate the "right side" of the expression (to the right of the equal sign).
- Once everything is figured out, place the computed value into the variables bucket.
We've already seen many examples of assignment. Assignment means: "storing a value (of a particular type) under a variable name" . Think of each assignment as copying the value of the righthand side of the expression into a "bucket" associated with the left hand side name!
Read this as, the variable called "name" is "assigned" the value computed by the expression to the right of the assignment operator ('=');
Now that you have seen some variables being assigned, tell me what the following code means?
The answer to above questions: the assignment means that lkjasdlfjlskdfjlksjdflkj is a variable (a really badly named one), but a variable none-the-less. jlkajdsf and lkjsdflkjsdf must also be variables. The sum of the two numbers held in jlkajdsf and lkjsdflkjsdf is stored in the variable lkjasdlfjlskdfjlksjdflkj.
Examples of builtin Data and Variables (and Constants)
For more info, use the "help" command: (e.g., help realmin);
Examples of using Data and Variable
Pattern to memorize, assignment pattern.
The assignment pattern creates a new variable, if this is the first time we have seen the "name", or, updates the variable to a new value!
Read the following code in English as: First, compute the value of the thing to the right of the assignment operator (the =). then store the computed value under the given name, destroying anything that was there before.
Or more concisely: assign the variable "name" the value computed by "right_hand_expression"

IMAGES
COMMENTS
An assignment operation is a process in imperative programming in which different values are associated with a particular variable name as time passes. [1] The program, in such model, operates by changing its state using successive assignment statements. [2] [3] Primitives of imperative programming languages rely on assignment to do iteration. [4]
The symbol used in an assignment statement is called as an operator. The symbol is ‘=’. Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’. The Basic Syntax of Assignment Statement in a programming language is : variable = expression ; where, variable = variable name
May 10, 2020 · There are some important properties of assignment in Python :-Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms ...
Three interrelated programming concepts are variables, declarations and assignment statements. Variables The concept of a variable is a powerful programming idea. It’s called a variable because – now pay attention – it varies. When you see it used in a program, the variable is often written like this r = 255; (r is the variable and the ...
The assignment statement 1 The assignment statement is used to store a value in a variable. As in most programming languages these days, the assignment statement has the form: <variable>= <expression>; For example, once we have an int variable j, we can assign it the value of expression 4 + 6: int j; j= 4+6;
Oct 23, 2023 · Assignment statements are essential in programming as they allow us to store and update data in variables. By using them, we can manipulate values, perform calculations, and create dynamic programs. So embrace the magic of assignment statements and let your code come to life! Happy coding in 2023 and beyond! 🚀
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements¶ Assignment statements initialize or change the value stored in a variable using the assignment operator =. An assignment statement always has a single variable on the left hand side.
Apr 25, 2024 · Assignment statements in a program come in two forms – with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. Assignments with mutation are variable assignments that use the old value of a variable to calculate a value for the variable. For example, an increment statement like x = x + 1 MUTATES the ...
ASSIGNMENT PATTERN . The assignment pattern creates a new variable, if this is the first time we have seen the "name", or, updates the variable to a new value! Read the following code in English as: First, compute the value of the thing to the right of the assignment operator (the =). then store the computed value under the given name ...
The first assignment statement puts A's value into C, making A=3, B=5 and C=3. The second assignment statements puts B's value into A. This destroys A's original value 3. After this, A = 5, B = 5 and C = 3. The third assignment statement puts C's value into B. This makes A=5, B=3 and C=3.